CSS의 아이디 혹은 클래스를 지정해두고 js로 해당 클래스 혹은 아이디에 접근한다.
id로 접근하는 방법
DOM (Document Object Module)
- js에서는 html파일 전체에 대한 부분을 document라는 객체로 받는다.
js에서 html파일에 접근할때는 document 객체를 사용해야한다.
- html 파일에 해당하는 부분에 id를 넣어준다.
html/css
<body>
<h1 id = "title">This works!</h1>
<script src = "index.js"></script>
</body>
#title {
background-color: green;
}
const getTitle = document.getElementById("title");
getTitle.innerHTML = "Hi! From JS";
getTitle은 document(HTML)에서 title이란 아이디를 가진 요소를 가져와서 넣었다.
즉, <h1 id = "title">이라는 부분을 js에서 getTitle로 변경하거나 접근할수 있다.
document 객체(HTML전체)에서 getElementById()함수를 이용해서 "title" 아이디를 가진요소를 getTitle에 넣었다.
getTitle(h1)의 객체로 h1안에 "Hi From JS" 문장을 넣었다.
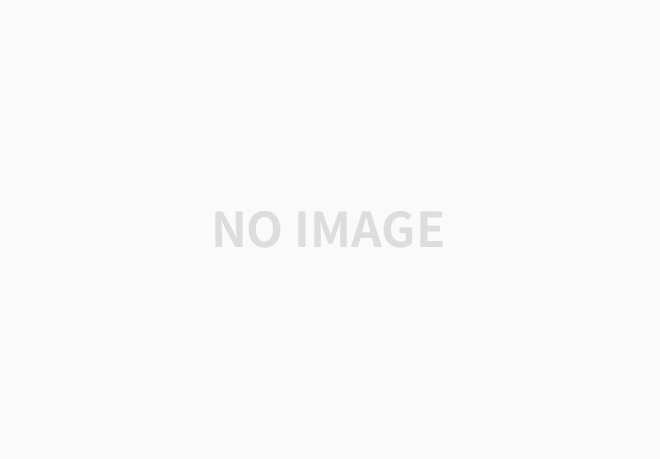
실제 html 코드로는 This works! 라고 작성되어 있음에도 웹브라우저 상에서는
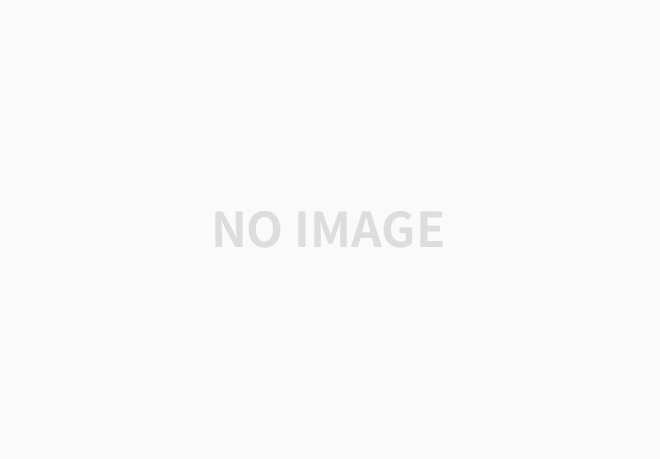
이렇게 바뀌어 있다. 이것이 JS!
즉, 내가 만든 페이지에서 모든 요소들은 JS에서 객체(DOM)가 된다.
그렇다면 이 객체 안에는 무엇이 들었을까?
아까 가져온 getTitle 안에 무엇이 들었는지 보자.
const getTitle = document.getElementById("title");
getTitle.innerHTML = "Hi! From JS";
console.dir(getTitle);
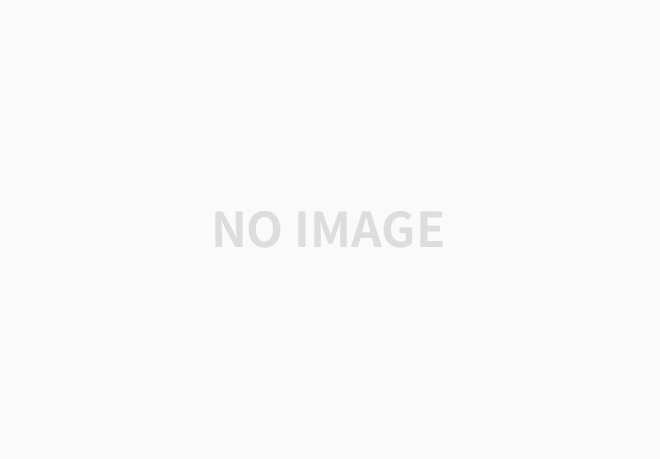
굉장히 많은 요소들이 들어있다.
이 요소들은 모두 내가 getTitle을 가지고 할수 있는 속성이다.
getTitle 말고도 document 의 속성들을 보자
console.dir(document);
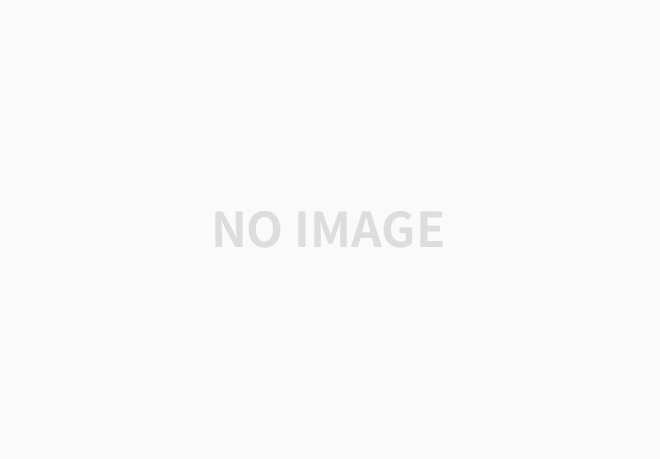
JS로 html 요소를 특정짓는 방법은 여러가지이다.
1. getElementById
2. getElementByClassName
3. getElemnetByTagName
4. querySelector("id이름, class이름")
- document의 모든 자식들 중에서 해당하는 이름이 있으면 반환한다.
console.dir(document);
// id가 title인 것을 찾을 때
let title = document.querySelector("#title");
// className이 title인 것을 찾을 때
let classTitle = document.querySelector(".title");
title.style.color = "green";
classTitle.innerHTML = "Hi classTitle";
어느 방법을 쓰든 객체를 받아온다는 점에서는 상관없는 것같다.
'Programming language > JavaScript' 카테고리의 다른 글
JavaScript - 5 [EventListener, if else 구문] (0) | 2020.11.11 |
---|---|
JavaScript - [VScode에서 Debugging 하기] (2) | 2020.11.10 |
JavaScript - 3 [Object, function] (0) | 2020.11.09 |
JavaScript - 2 [변수선언, String, float, int, Array] (1) | 2020.11.09 |
JavaScript - 1 [의미, 환경 설정, 실행] (4) | 2020.11.09 |